Recently I worked on a WordPress project that was undergoing a site refresh, and had frequent color changes. To save the hassle of having to go in and update colors for everything and also give the client control over the colors, I decided that the best course of action would be to make the colors available for admins. To save time on implementation and to keep things convenient for future changes, I decided to use the Customize API and map the values to CSS variables that can be used around the site.
What are CSS Variables?
CSS Variables are named variables that can have some value to be used in your CSS.
Below is a simple use-case, where we set a color to the CSS variables --color-primary
and use it as the background-color:
/* Global CSS Variables are set to :root*/
:root {
/* Set a color to button-color-primary */
--color-primary: #3c4b55;
}
.button {
/* Use var() to use a CSS Variable.*/
/* The second parameter takes a default value in case the variable is not set */
background-color: var(--color-primary, #000);
color: #ffffff;
}
CSS Variables allow you to prevent hard-coding properties that may need to be updated, and/or re-use values that should have the same value (ie. if buttons and cards should have the same background-color, they can use the same CSS variable to always have the same color).
Now with widespread browser support, CSS variables makes customizable UI elements very easy to integrate with CSS.
What is the WordPress Customizer (Customize API)?
The WordPress Customizer is one of many admin interfaces you can utilize to add settings/fields for your WordPress site. Unlike regular admin pages that require you to implement the interface and logic code from scratch, The Customize API provides a simple API to add fields with a few lines of code.
Below is an example on adding a simple color setting:
...
<?php
function setup_customizer( \WP_Customize_Manager $wp_customize ) {
// Create Custom Settings Section
$wp_customize->add_section( 'example_section', [
'title' => __('Custom Settings'),
'capability' => 'edit_theme_options',
] );
// Add Customizer Settings
$wp_customize->add_setting( 'example_color', [
'type' => 'theme_mod',
'capability' => 'edit_theme_options',
'default' => '#000000',
'transport' => 'refresh',
] );
// Create a Customizer Control (field) and attach to our section and setting above.
// Add Customizer Controls
$wp_customize->add_control( new \WP_Customize_Color_Control(
$wp_customize,
'example_color',
[
'label' => __('Example Color'),
'section' => 'example_section',
]
) );
}
...
Creating the Customizer Settings for CSS Variables
First we will use the Customize API to create our settings to set the color for a button background and color. To do that, we will need to hook into the customize_register
action:
<?php
/**
* Plugin Name: Customizer CSS Variable Example
*/
class CustomizerCSSVariableExample {
const SETTINGS_SECTION_ID = 'example_section';
public function __construct() {
add_action( 'customize_register', [$this, 'setup_customizer'], 10, 1 );
}
...
Next, we will setup a Customizer Section called “Custom Settings”, create two settings for the colors and add two color controls:
public function setup_customizer( \WP_Customize_Manager $wp_customize ) {
// Create Custom Settings Section
$wp_customize->add_section( self::SETTINGS_SECTION_ID, [
'title' => __('Custom Settings'),
'capability' => 'edit_theme_options',
] );
// Add Customizer Settings
$wp_customize->add_setting( 'example_button_color', [
'type' => 'theme_mod',
'capability' => 'edit_theme_options',
'default' => '#000000',
'transport' => 'refresh',
] );
$wp_customize->add_setting( 'example_button_text_color', [
'type' => 'theme_mod',
'capability' => 'edit_theme_options',
'default' => '#ffffff',
'transport' => 'refresh',
] );
// Add Customizer Controls
$wp_customize->add_control( new \WP_Customize_Color_Control(
$wp_customize,
'example_button_color',
[
'label' => __('Button Background Color'),
'section' => self::SETTINGS_SECTION_ID,
]
) );
$wp_customize->add_control( new \WP_Customize_Color_Control(
$wp_customize,
'example_button_text_color',
[
'label' => __('Button Text Color'),
'section' => self::SETTINGS_SECTION_ID,
]
) );
}
With that, our settings page is complete. It should look something like this:
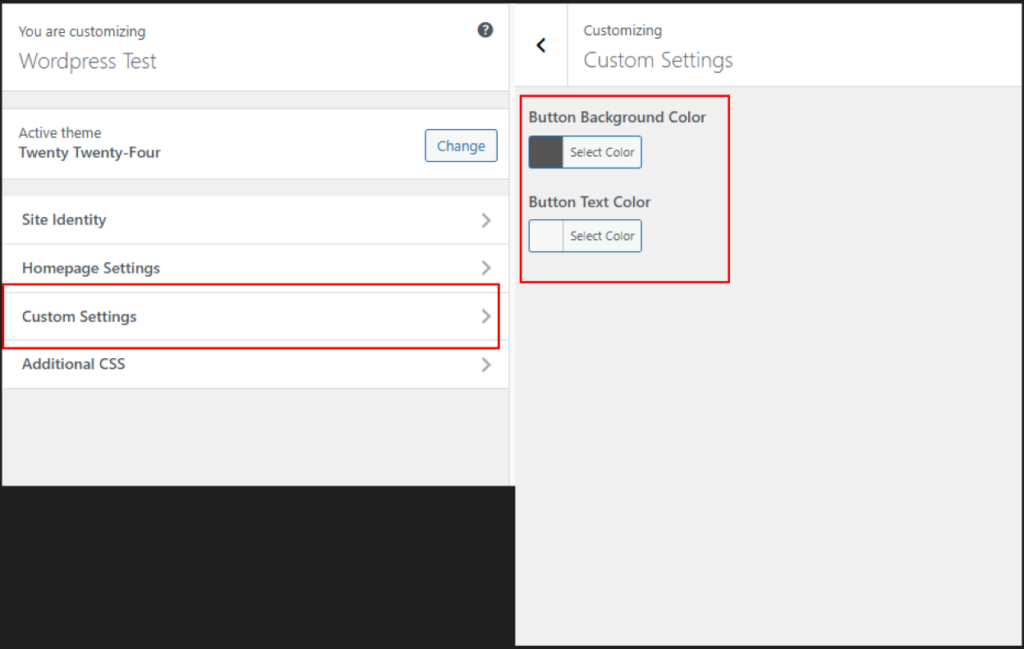
Creating CSS Variables Using the Customizer Settings
Now that the Customizer settings are completed, we will create CSS variables for them. We will define the CSS variables in wp_head
so we will need to hook into that. To make things easier, we will put all the variables we want to define into an array, and loop over that to output our CSS variables:
// Hooked into 'wp_head'
public function setup_css_variables() {
// Setup our variables.
// The array key name is the name of the variable
$css_variables = [
'example-button-bg-color' => get_theme_mod('example_button_color', '#000000'),
'example-button-text-color' => get_theme_mod('example_button_text_color', '#ffffff'),
];
// Output our variable in a <style> tag
// We loop through our array above to output all the css variables at once.
echo '<style>:root { ';
foreach( $css_variables as $_css_variable_name => $_css_variable_value ) {
echo "--{$_css_variable_name}: {$_css_variable_value};";
}
echo '}</style>';
}
With that, our CSS variables should be available on every page. To check, we can take a look at the page’s source code:

Awesome, now we can use the CSS Variables anywhere on the site.
To test, we will create a simple button
class in Theme > Customizer > Additional CSS.
.button {
background-color: var(--example-button-bg-color);
color: var(--example-button-text-color);
padding: 1rem 2rem;
border: 0;
border-radius: 3px;
}
We will use the class in a test page by using the Custom HTML Block and that’s it! We’re done.
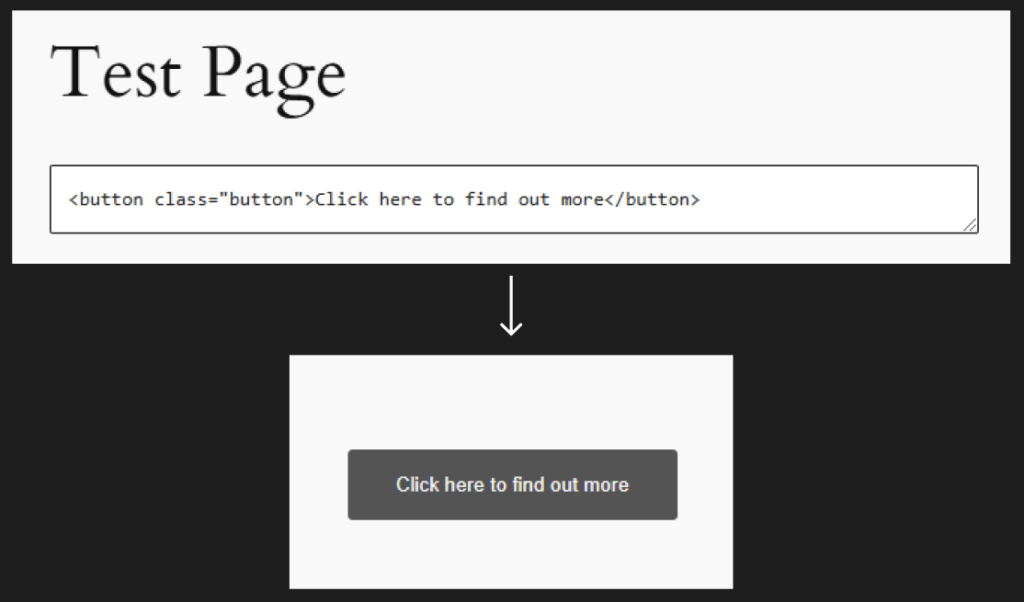
By utilizing the Customize API and CSS variables, you can quickly add settings in WordPress that automatically update the look and feel of your site. We used colors as an example, but it can be used for any CSS value ( background-image, spacing, content, etc.). CSS variables can also be overwritten through the same CSS Specificity rules, so it can be used to utilized to add default styling with custom styling on top, or specific stylings for certain UI components and so on.