When working on a WordPress site, I often find myself using WordPress’s command-line interface tool called WP-CLI. With WP-CLI you can do a lot of tasks that normally require a separate plugin to do, or tasks that normally may be challenging using just the website.
I will go over some of my most used commands in my day-to-day development.
WP-CLI Command: wp search-replace
Whenever it was time to launch a new site or go through site migration from one domain to another, there was always the requirement of replacing the domain names in the database. In the past, I have done things like a string replace directly in the database using SQL, or using plugins such as Better Search Replace to get it done. These methods always felt a bit cumbersome, but it did get the job done.
With the introduction of WP-CLI however, now we can do this easily via the command line with no additional plugin or setup.
Let’s say we have been working on a new site at example.dev
, and we are ready to launch it. All the site’s files and database have been migrated over, or perhaps we just want to update the domain. The new site will be example.com. We can accomplish this by using the search-replace
command:
wp search-replace 'https://example.dev' 'https://example.com'
This will go through the whole database, find https://example.dev
and replace it with https://example.com
. If you would like to be safe and do a test run without making any changes, you can use the --dry-run
option:
wp search-replace 'https://example.dev' 'https://example.com' --dry-run
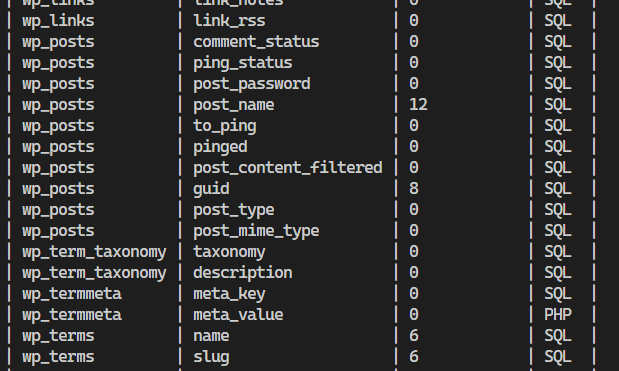
This is my biggest use case, however, the search-replace
command allows you to do much more involved operations such as search via regex using the --regex
option, export a SQL file instead of modifying the database using the --export
option, and more.
See the full documentation here
WP-CLI Command: wp profile
One of the more difficult things to do when working with PHP and WordPress is performance profiling. It is possible using xdebug, however setup is often difficult and confusing and sometimes cannot be done depending on the site’s setup. This is especially true if there is a performance issue on a production server.
This is where wp profile
comes in. It will measure the time taken by various parts of Worpress including core, plugins and the theme. It doesn’t provide a detailed nice view of your code that APM tools like Datadog or New Relic do, however, it does give you a pretty good idea of which part of the site may be taking a long time.
wp profile
is not part of the regular WP-CLI install and is an additional package. You can install it with the following command:
wp package install wp-cli/profile-command:@stable
Once installed, you can profile your site using
the stage
or hook
sub-commands.
wp profile stage
wp profile stage
can be used to get an overview of each different “stages” of WordPress’s initialization:

The three stages are:
- bootstrap: Initialization of WordPress, loading in the plugins and theme, and firing the
init
hook - main_query: Setting up the requested page’s main WP_Query
- template: Setting up and rendering the theme’s template
You can also dig a bit deeper into a specific stage if you want to see all the hooks fired there and how long they took by adding the stage name at the end. Below is an example output for wp profile stage bootstrap
:
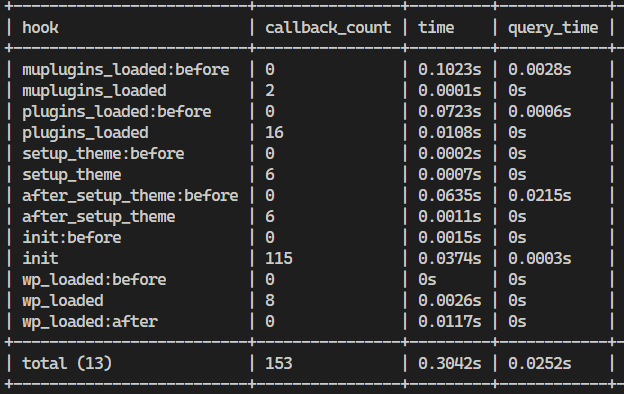
You can use wp profile stage
to find where you may be having performance problems. Once you find a hook you’re interested in, you can use the other sub-command:
wp profile hook
With wp profile hook <hook_name>
you can deep-dive into the specific function in the code that may be taking a bit too long. Let’s try looking into what is taking some time in the init
hook using wp profile hook init
. I will be sorting by time, descending by adding the following options:
wp profile hook init --orderby=time --order=DESC
The results look like this:

It looks like in this case, some plugins are using up most of the time. The great thing about this command is it tells you exactly what file and line number is causing the time.
If your site doesn’t have any APM or monitoring tools, this is a great option for profiling your site.
You can find out more about the profile command here.
WP-CLI Command: wp user
Occasionally as developers, we need to create new test users, or maybe we forgot our password and need to reset it. These operations are not exactly difficult on the site, but WP-CLI does make this simple.
Let’s say we started working on a site that is already existing. We have hosting access, SSH access, and database access however our client does not know how to create an admin user.
One way to create our user is to go into the database, INSERT
our new user into the wp_users
table, and set the proper capabilities. This is not too difficult, but it may take some time depending on the site’s hosting and setup. We can do it much easier with the wp user
command.
In the above example, if we wanted to create a new admin user, all we need to do is run the following:
wp user create example_admin [email protected] --role=administrator
This will create an administrator user with the username example_admin
. By default, a randomly generated password will be outputted to the console. If you want to specify your own password, you can use the --user_pass=<password>
option.
Another common situation is when you already have a user, but you forgot your password and want to change it. This can be achieved with wp user update
:
wp user update example_admin --user_pass=<password>
Now you can login with the new password!
Besides the above situations, the wp user
command has a lot of features such as updating roles/capabilities, updating user meta, auto user generation, and more.
You can see all of the options for wp user
here.
WP-CLI Command: wp cron
In a lot of projects, I usually have to implement and work with some type of scheduled tasks or features. Unfortunately, it is a bit difficult to work with WP Cron out of the box, as WordPress does not provide any admin features to work with WP Cron schedules.
Fortunately, WP-CLI provides the wp cron
command to do some basic operations with scheduled tasks.
Listing Scheduled Cron Events
To list schedule events, you can use the following command:
wp cron event list
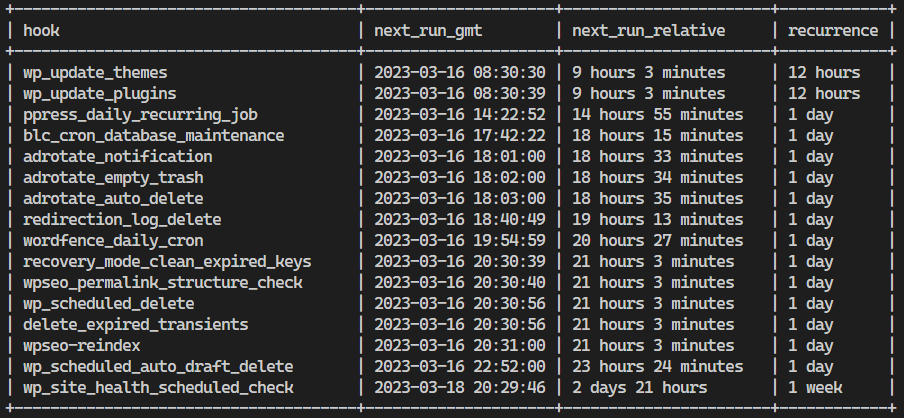
This will give you an overview of all of the scheduled events with their hook name, when it will next run, and the frequency.
Executing Scheduled Events
If you would like to execute a scheduled event, there are three options:
- wp cron event run <hook>: runs the event specified by hook right now.
- wp cron event run –due-now: runs all the events that are scheduled to be run.
- wp cron event run –all: runs all the scheduled events regardless of their scheduled next run.
The wp cron
command does not do a lot, but it is very helpful when working with WP Cron events.
See the full documentation here.
WP-CLI is a great tool allowing you to mostly do anything you can do in the admin section of WordPress and more in the command line. There are a lot more features and commands I did not cover here that can be used, with community-created packages like wp profile
that can be installed to expand its functionality. For those that are comfortable with the command line can even combine it with other server processes or scripts to automate tasks at the server level.
Click here to view all of the available commands for WP-CLI.
For packages to extend WP-CLI, you can check out the Package Index or search for WP-CLI packages on github.