In the WordPress 6.5 Update, WordPress introduced the Interactivity API. The Interactivity API allows “developers to create rich, interactive user experiences”. The main purpose for the Interactivity API is to provide a standard way for developers to develop interactive experiences when developing Gutenberg Blocks.
I like the idea of a standard, “WordPress way” of developing interactive experiences on the front-end. However there are still a lot of sites that do not use Gutenberg and blocks, or use custom, proprietary ways to implement the front-end.
In this post, we will explore how to implement the example on the Interactivity API Reference page as a plain old shortcode instead of a Gutenberg Block. Fortunately, it is fairly straight forward. The only main requirement is to add our own script as a script module, which will use the Interactivity API as a dependency.
The goal is to create a [clickme]
shortcode that will use the Interactivity API to work. In the examples below, I will be working with an empty test plugin. Similar methods should work fine in the theme’s functions.php.
Registering our main script via wp_register_script_module
Along with the Interactivity API, WordPress introduced wp_register_script_module()
, a function similar to wp_register_script()
, however this adds your script on the page as type="module"
. The Interactivity API script is registered as a script module, so we will need to add our own script via wp_register_script_module()
, with the Interactivity API as a dependency:
<?php
/**
* Plugin Name: Test Plugin
*/
namespace TestPlugin;
add_action('wp_enqueue_scripts', function() {
// Register our script as a script module
wp_register_script_module('clickme', plugins_url('/assets/js/clickme.js',__FILE__) , ['@wordpress/interactivity'], false);
});
It is possible to directly enqueue it via wp_enqueue_script_module()
, however that will load it on every page. So it is better practice to register it, and enqueue it only when it is used.
Registering our shortcode and setting up the HTML needed to use with the WordPress Interactivity API
First let’s go ahead and register our clickme
shortcode:
add_shortcode('clickme', function(array $attr = [], string $content = '', string $tag = '') {
wp_enqueue_script_module('clickme');
ob_start();
include __DIR__ . '/templates/clickme.php';
return ob_get_clean();
});
Here we are also enqueuing the script module registered above using wp_enqueue_script_module()
to make sure our script gets loaded when the shortcode is used.
The HTML is also organized into its own file, but this isn’t required.
clickme.php
<div
class="clickme"
data-wp-interactive="clickme"
data-wp-context='{"isOpen": false }'
data-wp-watch="callbacks.logIsOpen"
>
<button
data-wp-on--click="actions.toggle"
data-wp-bind--aria-expanded="context.isOpen"
aria-controls="p-1"
>
Toggle
</button>
<p id="p-1" data-wp-bind--hidden="!context.isOpen">
This element is now visible!
</p>
</div>
The contents of the template is similar to the “What are Directives?” section. The only difference is that we are using our own app name under the data-wp-interactive
attribute. With this, our shortcode should now render, but it is still not functional.
Creating and Setting up our main JS script to use with the WordPress Interactivity API
To implement the WordPress Interactivity API in javascript, all we need to do is to import the features we want from the Interactivity API and use it. The methods and contexts will be bound by the attributes defined in our template.
clickme.js
import { store, getContext } from "@wordpress/interactivity"
const { state } = store('clickme', {
state: {},
actions: {
toggle: () => {
const context = getContext();
context.isOpen = !context.isOpen;
}
},
callbacks: {
logIsOpen: () => {
const context = getContext();
console.log(`Is open: ${context.isOpen}`);
}
}
});
The main thing to keep in mind here is that the name is store()
must match the data-wp-interactive
directive in our template. If this is different, you will get an error.
And that’s it! The shortcode should display our little app which is utilizing the WordPress Interactivity API.
Shortcode in action (before and after clicked)
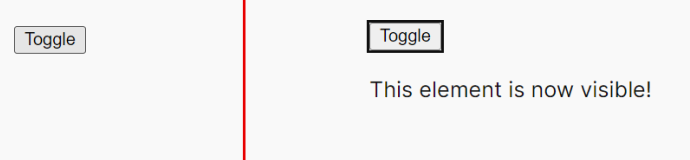