Overview
WordPress is a popular platform to use when developing websites. It is easy to spin up and deploy, easy to develop with, and most importantly, provides huge options for extensibility due to its large plugin and theme directory. WordPress and its plugins allow webmasters to add features and modify their websites even without any programming or development experience.
Unfortunately, not all plugins are properly optimized with the best web development practices in mind. A common issue amongst WordPress sites is that the site may start to use a lot of plugins, resulting in many unnecessary CSS styles and javascript loaded on every page load, even when they are not used on the particular page.
With increased assets, CSS styles, and javascript, the page load times get slower and slower. For sites that rely on SEO, this is very important now, especially with the introduction of Google’s Core Web Vitals (CWV). Core Web Vitals will determine whether your site or webpage meets Google’s page experience signal criteria.
To combat the issue of an evergrowing list of CSS styles and javascript, we will explore two different ways we can go about removing unused CSS styles and javascript from your WordPress pages.
Remove unused CSS styles and javascript using a plugin
The most popular plugin currently available to remove unused CSS styles and javascript from your WordPress pages is Asset CleanUp.
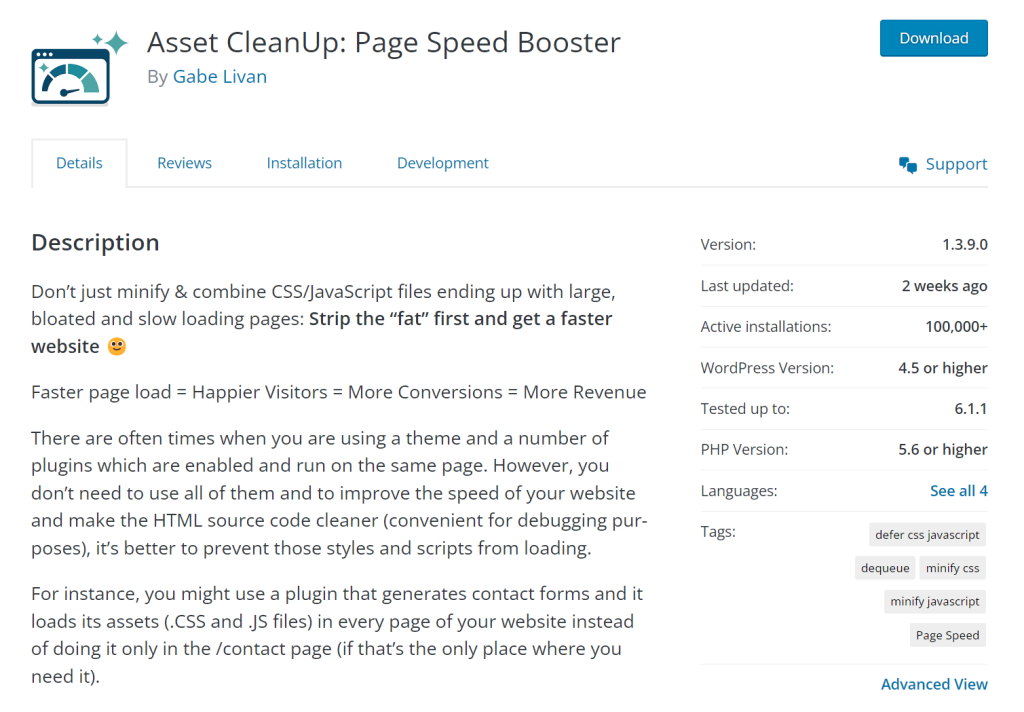
This plugin allows you to remove or “unload” CSS styles and javascript that other plugins automatically include on every page. Asset unloading can be done on a page-by-page basis, or site-wide.
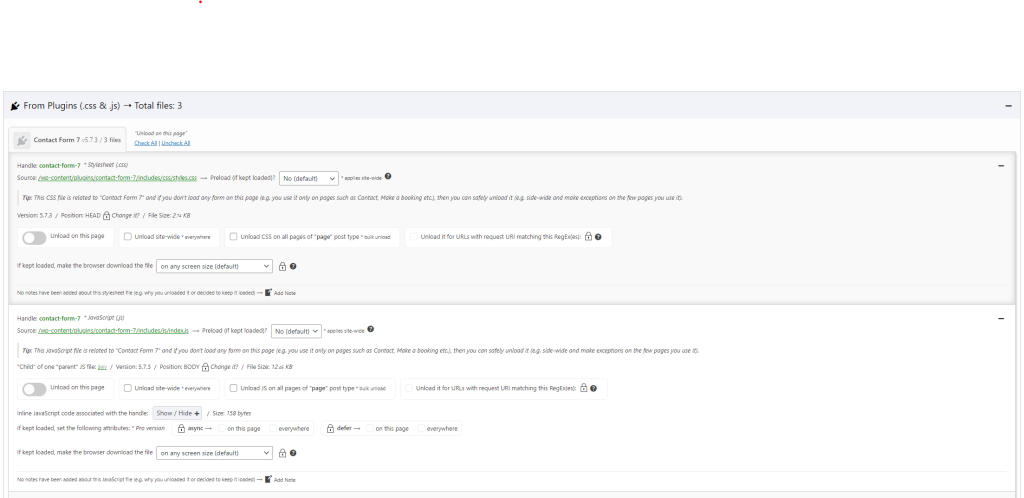
The plugin also allows you to unload theme and WordPress core assets, however, it is not recommended to unload those unless you know what you are doing. You are more likely to break the site’s look or feature.
Asset CleanUp is a great option if you are not a developer and/or there are many plugins to look through.
Remove unused CSS styles and javascript with code
The method above is easy and great, however, you are essentially adding yet another plugin to manage other plugin assets. If you would like to keep your plugin count low and/or manage removing unused assets yourself, you can do so with code using PHP.
Just as an example, let’s say our homepage does not have any forms, so we don’t want our Contact Form 7 assets loading there. We will go through disabling Contact Form 7’s assets from the homepage.
1. Find the asset’s handle
All CSS and javascript that are loaded in through WordPress properly goes through a function called wp_enqueue_script()
. This function takes a handle – an ID/name for the asset. The first step is to find out our assets handle. This can be done by looking through the plugin’s code, or an easier method is to use a plugin called Query Monitor. This plugin will list out all CSS and javascript that are loaded on a particular page via WordPress with their handle.

In this case, it looks like Contact Form 7 loads two different javascript files, with the handles being swv and contact-form-7. It loads one CSS file which also has the handle contact-form-7.
2. Call wp_dequeue_script()
and wp_dequeue_style
on the plugin’s assets
Now that we know the handles, we can proceed with the removal of the assets. We need somewhere to run the code, the easiest location is your theme’s functions.php
. Example is below:
functions.php
...
// WordPress action for loading CSS and javascript files
add_action( 'wp_enqueue_scripts', function() {
// Check if we're on the homepage
if( is_front_page() ) {
// Remove javascript files
wp_dequeue_script( 'swv' );
wp_dequeue_script( 'contact-form-7' );
// Remove CSS styling
wp_dequeue_style( 'contact-form-7' );
}
}, 99 );
...
With the above code in place, our homepage will no longer load the contact form 7 scripts, reducing the amount of assets loaded on our homepage. One plugin is not likely to make a big difference, but if you can find more assets that may be unneeded on certain pages it will add up to increased page load times.
Some plugins may include assets in non-standard ways, in which case this method will not work. Some assets may also require you to use wp_deregister_script()
and wp_deregister_style()
. You may also need to use the wp_print_scripts
action if wp_enqueue_scripts
is too early. Some trial and error may be needed to get the best results depending on the plugin.